|
楼主 |
发表于 2009-1-16 05:31:04
|
显示全部楼层
来自 中国–广东–广州–白云区
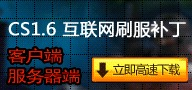
- // restore speed and remove glow
- new speed = floatround(get_user_maxspeed(id) / (get_cvar_float("fn_chill_speed") / 100.0));
- set_user_maxspeed(id,float(speed));
- set_user_rendering(id);
- // kill their trail
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(99); // TE_KILLBEAM
- write_short(id);
- message_end();
- }
- }
- // a player's freeze runs out
- public remove_freeze(taskid)
- {
- remove_task(taskid);
- new id = taskid - TASK_REMOVE_FREEZE;
- // no longer frozen
- if(!isFrozen[id])
- return;
- // if nothing happened to the model
- if(is_valid_ent(novaDisplay[id]))
- {
- // get origin of their frost nova
- new origin[3], Float:originF[3];
- entity_get_vector(novaDisplay[id],EV_VEC_origin,originF);
- FVecIVec(originF,origin);
- // add some tracers
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(14); // TE_IMPLOSION
- write_coord(origin[0]); // x
- write_coord(origin[1]); // y
- write_coord(origin[2] + 8); // z
- write_byte(34); // radius
- write_byte(10); // count
- write_byte(3); // duration
- message_end();
- // add some sparks
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(9); // TE_SPARKS
- write_coord(origin[0]); // x
- write_coord(origin[1]); // y
- write_coord(origin[2]); // z
- message_end();
- // add the shatter
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(108); // TE_BREAKMODEL
- write_coord(origin[0]); // x
- write_coord(origin[1]); // y
- write_coord(origin[2] + 24); // z
- write_coord(16); // size x
- write_coord(16); // size y
- write_coord(16); // size z
- write_coord(random_num(-50,50)); // velocity x
- write_coord(random_num(-50,50)); // velocity y
- write_coord(25); // velocity z
- write_byte(10); // random velocity
- write_short(glassGibs); // model
- write_byte(10); // count
- write_byte(25); // life
- write_byte(0x01); // flags: BREAK_GLASS
- message_end();
- // play a sound and remove the model
- emit_sound(novaDisplay[id],CHAN_BODY,"warcraft3/impalelaunch1.wav",1.0,ATTN_NORM,0,PITCH_LOW);
- remove_entity(novaDisplay[id]);
- }
- isFrozen[id] = 0;
- novaDisplay[id] = 0;
- // only apply effects to this player if they are still connected
- if(is_user_connected(id))
- {
- // restore gravity
- entity_set_float(id,EV_FL_gravity,1.0);
- // if they are still chilled, set the speed rightly so. otherwise, restore it to complete regular.
- if(isChilled[id])
- {
- set_beamfollow(id,30,8,FROST_R,FROST_G,FROST_B,100); // bug fix
- new speed = floatround(oldSpeed[id] * (get_cvar_float("fn_chill_speed") / 100.0));
- set_user_maxspeed(id,float(speed));
- }
- else
- set_user_maxspeed(id,oldSpeed[id]);
- }
- oldSpeed[id] = 0.0;
- }
- /*----------------
- UTILITY FUNCTIONS
- -----------------*/
- // my own radius calculations...
- //
- // 1. figure out how far a player is from a center point
- // 2. figure the percentage this distance is of the overall radius
- // 3. find a value between maxVal and minVal based on this percentage
- //
- // example: origin1 is 96 units away from origin2, and radius is 240.
- // this player is then 60% towards the center from the edge of the sphere.
- // let us say maxVal is 100.0 and minVal is 25.0. 60% progression from minimum
- // to maximum becomes 70.0. tada!
- public Float:radius_calucation(Float:origin1[3],Float:origin2[3],Float:radius,Float:maxVal,Float:minVal)
- {
- if(maxVal <= 0.0)
- return 0.0;
- if(minVal >= maxVal)
- return minVal;
- new Float:percent;
- // figure out how far away the points are
- new Float:distance = vector_distance(origin1,origin2);
- // if we are close enough, assume we are at the center
- if(distance < 40.0)
- return maxVal;
- // otherwise, calculate the distance range
- else
- percent = 1.0 - (distance / radius);
- // we have the technology...
- return minVal + (percent * (maxVal - minVal));
- }
- // displays x y z axis
- public show_xyz(origin[3],radius)
- {
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(0); // TE_BEAMPOINTS
- write_coord(origin[0]); // start x
- write_coord(origin[1]); // starty
- write_coord(origin[2] + 1); // start z
- write_coord(origin[0] + radius); // end x
- write_coord(origin[1]); // end y
- write_coord(origin[2] + 1); // end z
- write_short(trailSpr); // sprite
- write_byte(0); // starting frame
- write_byte(0); // framerate
- write_byte(50); // life
- write_byte(10); // line width
- write_byte(0); // noise
- write_byte(255); // r
- write_byte(0); // g
- write_byte(0); // b
- write_byte(200); // brightness
- write_byte(0); // scroll speed
- message_end();
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(0); // TE_BEAMPOINTS
- write_coord(origin[0]); // start x
- write_coord(origin[1]); // starty
- write_coord(origin[2] + 1); // start z
- write_coord(origin[0]); // end x
- write_coord(origin[1] + radius); // end y
- write_coord(origin[2] + 1); // end z
- write_short(trailSpr); // sprite
- write_byte(0); // starting frame
- write_byte(0); // framerate
- write_byte(50); // life
- write_byte(10); // line width
- write_byte(0); // noise
- write_byte(0); // r
- write_byte(255); // g
- write_byte(0); // b
- write_byte(200); // brightness
- write_byte(0); // scroll speed
- message_end();
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(0); // TE_BEAMPOINTS
- write_coord(origin[0]); // start x
- write_coord(origin[1]); // starty
- write_coord(origin[2]); // start z
- write_coord(origin[0]); // end x
- write_coord(origin[1]); // end y
- write_coord(origin[2] + radius); // end z
- write_short(trailSpr); // sprite
- write_byte(0); // starting frame
- write_byte(0); // framerate
- write_byte(50); // life
- write_byte(10); // line width
- write_byte(0); // noise
- write_byte(0); // r
- write_byte(0); // g
- write_byte(255); // b
- write_byte(200); // brightness
- write_byte(0); // scroll speed
- message_end();
- }
- // give an entity a trail
- public set_beamfollow(ent,life,width,r,g,b,brightness)
- {
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(22); // TE_BEAMFOLLOW
- write_short(ent); // ball
- write_short(trailSpr); // sprite
- write_byte(life); // life
- write_byte(width); // width
- write_byte(r); // r
- write_byte(g); // g
- write_byte(b); // b
- write_byte(brightness); // brightness
- message_end();
- }
- // blue blast
- public create_blast(origin[3])
- {
- // smallest ring
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(21); // TE_BEAMCYLINDER
- write_coord(origin[0]); // start X
- write_coord(origin[1]); // start Y
- write_coord(origin[2]); // start Z
- write_coord(origin[0]); // something X
- write_coord(origin[1]); // something Y
- write_coord(origin[2] + 385); // something Z
- write_short(exploSpr); // sprite
- write_byte(0); // startframe
- write_byte(0); // framerate
- write_byte(12); // life
- write_byte(50); // width
- write_byte(0); // noise
- write_byte(FROST_R); // red
- write_byte(FROST_G); // green
- write_byte(FROST_B); // blue
- write_byte(50); // brightness
- write_byte(0); // speed
- message_end();
- // medium ring
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(21); // TE_BEAMCYLINDER
- write_coord(origin[0]); // start X
- write_coord(origin[1]); // start Y
- write_coord(origin[2]); // start Z
- write_coord(origin[0]); // something X
- write_coord(origin[1]); // something Y
- write_coord(origin[2] + 470); // something Z
- write_short(exploSpr); // sprite
- write_byte(0); // startframe
- write_byte(0); // framerate
- write_byte(12); // life
- write_byte(50); // width
- write_byte(0); // noise
- write_byte(FROST_R); // red
- write_byte(FROST_G); // green
- write_byte(FROST_B); // blue
- write_byte(50); // brightness
- write_byte(0); // speed
- message_end();
- // largest ring
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(21); // TE_BEAMCYLINDER
- write_coord(origin[0]); // start X
- write_coord(origin[1]); // start Y
- write_coord(origin[2]); // start Z
- write_coord(origin[0]); // something X
- write_coord(origin[1]); // something Y
- write_coord(origin[2] + 555); // something Z
- write_short(exploSpr); // sprite
- write_byte(0); // startframe
- write_byte(0); // framerate
- write_byte(12); // life
- write_byte(50); // width
- write_byte(0); // noise
- write_byte(FROST_R); // red
- write_byte(FROST_G); // green
- write_byte(FROST_B); // blue
- write_byte(50); // brightness
- write_byte(0); // speed
- message_end();
- // light effect
- message_begin(MSG_BROADCAST,SVC_TEMPENTITY);
- write_byte(27); // TE_DLIGHT
- write_coord(origin[0]); // x
- write_coord(origin[1]); // y
- write_coord(origin[2]); // z
- write_byte(floatround(FROST_RADIUS/5.0)); // radius
- write_byte(FROST_R); // r
- write_byte(FROST_G); // g
- write_byte(FROST_B); // b
- write_byte(20); // life
- write_byte(30); // decay rate
- message_end();
- }
复制代码 40# 点通粉丝 |
|