|
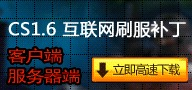 L 02/22/2005 - 21:41:34: [AMXX] Debug Trace =>
L 02/22/2005 - 21:41:34: [AMXX] [0] Line 120, File "anticamping_x.sma"
L 02/22/2005 - 21:41:34: [AMXX] [1] Line 278, File "anticamping_x.sma"
L 02/22/2005 - 21:41:34: [AMXX] Run time error 25 (parameter error) on line 120 (plugin "anticamping_x.amxx").
L 02/22/2005 - 21:41:34: String formatted incorrectly - parameter 3 (total 2)
- /* AMX Mod script
- *
- * AntiCamping Advanced
- * by Homicide, original code by SpaceDude
- *
- * This script is a modification to SpaceDude's anti-camping plugin.
- * I used Spacedudes method for determine camping then added some nice features to the plugin.
- *
- * The main feature I added was the heartbeat method of discouraging camping.
- *
- * The features include the ability to set punishment, camping time, healthpunish, and toggle 'the campmeter'.
- *
- * Cvars:
- * anticamping 0|1 蹲坑检测 0=关闭, 1=开启 | 默认: 1
- * anticamping_time n 允许蹲坑的时间上限 | 默认: 20
- * anticamping_punish_mode abcde 蹲坑惩罚的方式, a=扇耳光, b=减少生命值, c=举牌子, d=呼吸声, e=亮人, 可复选 | 默认:bc
- * anticamping_healthpunish n 每次减少生命值的数量(如果选择了减少生命的惩罚方式) | 默认: 10
- * anticamping_meter 0|1 向客户端显示检测的信息, 0=关闭, 1=开启 | 默认: 1
- * anticamping_camp_limit n 每个玩家的蹲坑限次 | 默认: 3
- * anticamping_kick 0|1 是否自动踢出超过蹲坑限次的玩家, 1=开启, 0=关闭 | 默认: 1
- *
- * note: this plugin is best used without any other anti-camp plugins
- * origin auth:Homicide- original code by SpaceDude
- * edit by nwb13
- */
- #include <amxmodx>
- #include <fun>
- #define SND_STOP (1<<5)
- #define TASK_CAMP 6666667
- #define DETECT_RANGE 30.0 // 检测范围
- #define ROW_NUM 5 // 进度条的数量
- new playercoord0[33][3]
- new playercoord1[33][3]
- new playercoord2[33][3]
- new playercoord3[33][3]
- new playercoord4[33][3]
- new campmeter[33]
- new g_pl_camptimes[33] // 玩家的蹲坑次数
- new bool:pausecounter[33]
- new bool:bombplanted
- new bool:de_map
- new camptolerancedefending = 180
- new camptoleranceattacking = 200
- new bool:g_pl_spr[33] // 玩家的牌子状态
- new bool:g_pl_lim[33] // 玩家蹲坑到100%的状态
- new camper_sprite
- new cvarflags[8] // 惩罚方式
- new bool:g_pl_dtp[33] // 检测玩家死亡时是否是正在蹲坑
- new bool:g_pl_glow[33] // 检测玩家是否被亮人
- public plugin_init() {
- register_plugin("AntiCamping Advanced X","0.1","nwb13")
- register_event("Damage", "damage_event", "b", "2!0")
- register_event("BarTime","bartime_event","b")
- register_event("ResetHUD", "new_round", "b")
- register_event("SendAudio", "bomb_planted", "a", "2&%!MRAD_BOMBPL")
- register_event("SendAudio", "round_end", "a", "2&%!MRAD_terwin","2&%!MRAD_ctwin","2&%!MRAD_rounddraw")
- register_event("StatusIcon", "got_bomb", "be", "1=1", "1=2", "2=c4")
- register_event("CS_DeathMsg", "on_death", "a")
- register_cvar("anticamping","1") // 蹲坑检测 0=关闭, 1=开启
- register_cvar("anticamping_camptime","20") // 允许蹲坑的时间上限
- register_cvar("anticamping_punish_mode","bc") // 对蹲坑玩家的惩罚方式, a=扇耳光, b=减少生命值, c=举牌子, d=呼吸声, e=亮人
- register_cvar("anticamping_healthpunish","10") // 每次减少生命值的数量
- register_cvar("anticamping_meter","1") // 向客户端显示检测的信息, 0=关闭, 1=开启
- register_cvar("anticamping_camp_limit","3") // 每个玩家的蹲坑限次
- register_cvar("anticamping_kick","1") // 是否自动踢出超过蹲坑限次的玩家, 1=开启, 0=关闭(但是立即杀死玩家并减上限数1)
- register_cvar("anticamping_de_map_allow","1") //de_地图上是否允许防守方蹲坑
- set_task(1.0,"checkcamping",1)
- return PLUGIN_CONTINUE
- }
- public sqrt(num) {
- new div = num;
- new result = 1;
- while (div > result) { // end when div == result, or just below
- div = (div + result) / 2 // take mean value as new divisor
- result = num / div
- }
- return div;
- }
- public unpausecounter(parm[]) {
- new id = parm[0]
- pausecounter[id] = false
- return PLUGIN_CONTINUE
- }
- public displaymeter(id) {
- if (get_cvar_num("anticamping_meter") != 0) {
- new buffer[128],temp,pos
- if ((get_user_team(id) == 1) && bombplanted && de_map && get_cvar_num("anticamping_de_map_allow"))
- pos += format(buffer[pos],127-pos,"允许蹲坑以防止警察拆解炸弹")
- else if ((get_user_team(id) == 2) && (!bombplanted) && de_map && get_cvar_num("anticamping_de_map_allow"))
- pos += format(buffer[pos],127-pos,"允许蹲坑以防止匪徒布置炸弹")
- else {
- temp = campmeter[id]/ROW_NUM
- for (new x=0; x<temp; x++)
- pos += format(buffer[pos],127-pos,"|")
- for (new y=0; y<(100/ROW_NUM-temp); y++)
- pos += format(buffer[pos],127-pos,"_")
- pos += format(buffer[pos],127-pos,"^n蹲坑状态: %i%% 蹲坑次数: %d/%d",campmeter[id],g_pl_camptimes[id],get_cvar_num("anticamping_camp_limit"))
- }
- if (campmeter[id] > 100) {
- set_hudmessage(255, 0, 0, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- } else if (campmeter[id] > 90) {
- set_hudmessage(255, 0, 0, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- } else if (campmeter[id] > 80){
- set_hudmessage(255, 100, 0, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- } else if (campmeter[id] > 50 ) {
- set_hudmessage(255, 255, 0, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- } else if (campmeter[id] > 20 ) {
- set_hudmessage(0, 255, 0, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- } else {
- set_hudmessage(255, 255, 255, 0.02, 0.87, 0, 1.0, 2.0, 0.1, 0.1, 4)
- }
- show_hudmessage(id,buffer)
- }
- return PLUGIN_HANDLED
- }
- public checkcamping(){
- if (!get_cvar_num("anticamping")){
- set_task(1.0,"checkcamping",1)
- return PLUGIN_CONTINUE
- }
- new players[32],variance[3],average[3]
- new numberofplayers, variancetotal, standarddeviation, id, i, j, team
- get_cvar_string("anticamping_punish_mode", cvarflags, 8)
- get_players(players, numberofplayers, "a")
- for (i = 0; i < numberofplayers; ++i) {
- while (i < numberofplayers && pausecounter[players[i]]) {
- ++i
- }
- if (i >= numberofplayers){
- set_task(1.0,"checkcamping",1)
- return PLUGIN_CONTINUE
- }
- id = players[i]
- for (j = 0; j < 3; ++j) {
- playercoord4[id][j] = playercoord3[id][j]
- playercoord3[id][j] = playercoord2[id][j]
- playercoord2[id][j] = playercoord1[id][j]
- playercoord1[id][j] = playercoord0[id][j]
- }
- get_user_origin(id, playercoord0[id], 0)
- for (j = 0; j < 3; ++j) {
- average[j] = (playercoord0[id][j] +
- playercoord1[id][j] +
- playercoord2[id][j] +
- playercoord3[id][j] +
- playercoord4[id][j]) / 5
- variance[j] = (((playercoord0[id][j] - average[j]) * (playercoord0[id][j] - average[j]) +
- (playercoord1[id][j] - average[j]) * (playercoord1[id][j] - average[j]) +
- (playercoord2[id][j] - average[j]) * (playercoord2[id][j] - average[j]) +
- (playercoord3[id][j] - average[j]) * (playercoord3[id][j] - average[j]) +
- (playercoord4[id][j] - average[j]) * (playercoord4[id][j] - average[j])) / 4)
- }
- variancetotal=variance[0]+variance[1]+variance[2]
- standarddeviation=sqrt(variancetotal)
- team = get_user_team(id)
- if (!de_map){
- if (team == 2) // Team 1 = Terro, Team 2 = CT
- campmeter[id] += (camptoleranceattacking - standarddeviation) / get_cvar_num("anticamping_camptime")
- else
- campmeter[id] += (camptoleranceattacking - standarddeviation) / get_cvar_num("anticamping_camptime")
- }
- else if (bombplanted){
- if (team == 1) // Team 1 = Terro, Team 2 = CT
- if (get_cvar_num("anticamping_de_map_allow"))
- campmeter[id] = 0
- else
- campmeter[id] += (camptolerancedefending - standarddeviation) / get_cvar_num("anticamping_camptime")
- else
- campmeter[id] += (camptoleranceattacking - standarddeviation) / get_cvar_num("anticamping_camptime")
- }
- else{
- if (team == 2) // Team 1 = Terro, Team 2 = CT
- if (get_cvar_num("anticamping_de_map_allow"))
- campmeter[id] = 0
- else
- campmeter[id] += (camptolerancedefending - standarddeviation) / get_cvar_num("anticamping_camptime")
- else
- campmeter[id] += (camptoleranceattacking - standarddeviation) / get_cvar_num("anticamping_camptime")
- }
- if (g_pl_camptimes[id] >= get_cvar_num("anticamping_camp_limit")) {
- switch (get_cvar_num("anticamping_kick")){
- case 0:{
- client_print(id,print_chat,"* 你蹲坑的次数达到了上限, 所以立即处死你以示警告!")
- user_slap(id,get_user_health(id))
- g_pl_camptimes[id]--
- }
- case 1:{
- client_cmd(id,"echo ^"该服务器讨厌爱蹲坑的玩家^"")
- server_cmd("kick #%d ^"你达到了蹲坑限次, 被踢出服务器^"", get_user_userid(id))
- }
- }
- }
- if ((campmeter[id] < 80 ) && is_user_connected(id)) {
- if (g_pl_lim[id] == true){
- g_pl_lim[id] = false
- }
- if ((contain(cvarflags,"c") != -1) && (g_pl_spr[id] == true)) {
- new parm[2]
- parm[0] = id
- parm[1] = 0
- set_task(0.5,"clear_spr",TASK_CAMP+id,parm,2)
- }
- if ((contain(cvarflags,"e") != -1) && g_pl_glow[id]) {
- set_user_rendering(id,kRenderFxNone,255,255,255,kRenderNormal,1)
- g_pl_glow[id] = false
- }
- if (contain(cvarflags,"d") != -1) {
- emit_sound(id,CHAN_VOICE,"player/breathe2.wav", 0.0, ATTN_NORM, SND_STOP, PITCH_NORM)
- }
- }
- if ((campmeter[id] < 0) && is_user_connected(id)){
- campmeter[id] = 0
- } else if ((campmeter[id]>100) && is_user_connected(id)) {
- if (contain(cvarflags,"a") != -1) {
- user_slap(id,get_cvar_num("anticamping_healthpunish"))
- }
- if (contain(cvarflags,"b") != -1) {
- set_user_health(id, get_user_health(id) - get_cvar_num("anticamping_healthpunish"))
- }
- if ((contain(cvarflags,"c") != -1) && (g_pl_spr[id] == false)) {
- new parm[1]
- parm[0] = id
- set_task(0.5,"show_camper_spr",TASK_CAMP+id,parm,1)
- }
- if (contain(cvarflags,"d") != -1) {
- emit_sound(id,CHAN_VOICE,"player/breathe2.wav", 1.0, ATTN_NORM, 0, PITCH_NORM)
- }
- if (contain(cvarflags,"e") != -1) {
- new colour_r,colour_b
- if (team == 1){
- colour_r = 255
- colour_b = 0
- }else{
- colour_r = 0
- colour_b = 255
- }
- set_user_rendering(id,kRenderFxGlowShell,colour_r,0,colour_b,kRenderNormal,17)
- g_pl_glow[id] = true
- }
- if (g_pl_lim[id] == false){
- g_pl_camptimes[id]++
- detect_enemy(id)
- g_pl_lim[id] = true
- }
- campmeter[id] = 100
- } else if ((campmeter[id] > 90) && is_user_connected(id)) {
- if (contain(cvarflags,"a") != -1) {
- user_slap(id,get_cvar_num("anticamping_healthpunish") / 5)
- }
- if (contain(cvarflags,"b") != -1) {
- set_user_health(id, get_user_health(id) - get_cvar_num("anticamping_healthpunish") / 5)
- }
- if (contain(cvarflags,"d") != -1) {
- emit_sound(id,CHAN_VOICE,"player/breathe2.wav", 0.5, ATTN_NORM, 0, PITCH_NORM)
- }
- } else if ((campmeter[id]>80) && is_user_connected(id)) {
- if (contain(cvarflags,"a") != -1) {
- user_slap(id,get_cvar_num("anticamping_healthpunish") / 10)
- }
- if (contain(cvarflags,"b") != -1) {
- set_user_health(id, get_user_health(id) - get_cvar_num("anticamping_healthpunish") / 10)
- }
- if (contain(cvarflags,"d") != -1) {
- emit_sound(id,CHAN_VOICE,"player/breathe2.wav", 0.1, ATTN_NORM, 0, PITCH_NORM)
- }
- }
- if (is_user_connected(id) && get_cvar_num("anticamping"))
- displaymeter(id)
- }
- set_task(1.5,"checkcamping",1)
- return PLUGIN_CONTINUE
- }
- public damage_event(id) {
- if (get_cvar_num("anticamping")) {
- new enemy = get_user_attacker(id)
- if (get_user_team(id)!=get_user_team(enemy)) {
- campmeter[id]=0
- campmeter[enemy]=0
- }
- return PLUGIN_CONTINUE
- }
- return PLUGIN_CONTINUE
- }
- public new_round(id) {
- if (get_cvar_num("anticamping")) {
- bombplanted=false
- pausecounter[id]=true
- campmeter[id]=0
- new Float:freezetime = get_cvar_float("mp_freezetime")+1.0
- new parm[1]
- parm[0]=id
- set_task(freezetime,"unpausecounter",0,parm,1)
- return PLUGIN_CONTINUE
- }
- return PLUGIN_CONTINUE
- }
- public bartime_event(id) {
- if (get_cvar_num("anticamping")) {
- pausecounter[id]=true
- campmeter[id]=0
- new Float:bar_time=float(read_data(1)+1)
- new parm[1]
- parm[0]=id
- set_task(bar_time,"unpausecounter",0,parm,1)
- return PLUGIN_CONTINUE
- }
- return PLUGIN_CONTINUE
- }
- public bomb_planted() {
- if (get_cvar_num("anticamping")) {
- bombplanted=true
- return PLUGIN_CONTINUE
- }
- return PLUGIN_CONTINUE
- }
- public got_bomb(id) {
- if (get_cvar_num("anticamping")) {
- de_map=true
- return PLUGIN_CONTINUE
- }
- return PLUGIN_CONTINUE
- }
- public round_end() {
- if (get_cvar_num("anticamping")) {
- new players[32]
- new numberofplayers
- new id
- new i
- get_players(players, numberofplayers, "a")
- for (i = 0; i < numberofplayers; ++i) {
- id=players[i]
- //pausecounter[id]=true
- campmeter[id]=0
- return PLUGIN_CONTINUE
- }
- }
- return PLUGIN_CONTINUE
- }
- public plugin_precache() {
- precache_sound("player/breathe2.wav")
- camper_sprite = precache_model("sprites/camper.spr")
- return PLUGIN_CONTINUE
- }
- public show_camper_spr(parm[]) {
- new id = parm[0]
- message_begin(MSG_ALL,SVC_TEMPENTITY)
- write_byte(124)
- write_byte(id)
- write_coord(65)
- write_short(camper_sprite)
- write_short(32767)
- message_end()
- g_pl_spr[id] = true
- show_camp_message(id,1)
- return PLUGIN_CONTINUE
- }
- show_camp_message(id,type) {
- new wps[32],buffer[512],name[32],wpnname[32]
- new num,clip,ammo,pos
- new health = get_user_health(id)
- new armor = get_user_armor(id)
- get_user_name(id,name,31)
- if (type == 1){
- pos += format(buffer[pos],511-pos,"警告: %s (HP %d AP %d) 已长时间蹲坑^n他配备的武器及弹药量:^n",name,health,armor)
- }else{
- pos += format(buffer[pos],511-pos,"警告: %s (HP %d AP %d) 离开了蹲坑点^n他配备的武器及弹药量:^n",name,health,armor)
- }
- get_user_weapons(id,wps,num)
- for (new i=0; i<num; i++) {
- get_wpname(wps[i],wpnname,31)
- get_user_ammo(id, wps[i], ammo, clip)
- if (ammo < 0) ammo = 0
- if (clip < 0) clip = 0
- if (wps[i] == CSW_C4 || wps[i] == CSW_HEGRENADE || wps[i] == CSW_SMOKEGRENADE || wps[i] == CSW_FLASHBANG )
- pos += format(buffer[pos],511-pos,"%s %d 枚^n",wpnname,clip)
- else if (wps[i] == CSW_KNIFE )
- pos += format(buffer[pos],511-pos,"%s^n",wpnname)
- else
- pos += format(buffer[pos],511-pos,"%s 弹夹%d发/备用%d发^n",wpnname,ammo,clip)
- }
- if (type == 1){
- pos += format(buffer[pos],511-pos,"请大家搜捕并歼灭他!")
- }else{
- pos += format(buffer[pos],511-pos,"大家请留神任何可蹲坑的地点.")
- }
- if (type == 1)
- set_hudmessage(230, 180, 0, 0.17, 0.05, 0, 6.0, 10.0, 0.1, 0.15, 3)
- else
- set_hudmessage(154, 20, 255, 0.17, 0.05, 0, 6.0, 10.0, 0.1, 0.15, 3)
- show_hudmessage(0,buffer)
- }
- get_wpname(wp, name[], imax) {
- switch (wp) {
- case CSW_P228:
- copy(name, imax, "P228")
- case CSW_SCOUT:
- copy(name, imax, "Scout")
- case CSW_HEGRENADE:
- copy(name, imax, "高爆手雷")
- case CSW_XM1014:
- copy(name, imax, "Xm1014")
- case CSW_C4:
- copy(name, imax, "炸药包")
- case CSW_MAC10:
- copy(name, imax, "Mac10")
- case CSW_AUG:
- copy(name, imax, "Aug")
- case CSW_SMOKEGRENADE:
- copy(name, imax, "烟雾雷")
- case CSW_ELITE:
- copy(name, imax, "Elite")
- case CSW_FIVESEVEN:
- copy(name, imax, "Fiveseven")
- case CSW_UMP45:
- copy(name, imax, "Ump45")
- case CSW_SG550:
- copy(name, imax, "Sg550")
- case CSW_GALIL:
- copy(name, imax, "Galil")
- case CSW_FAMAS:
- copy(name, imax, "Famas")
- case CSW_USP:
- copy(name, imax, "Usp")
- case CSW_GLOCK18:
- copy(name, imax, "Glock18")
- case CSW_AWP:
- copy(name, imax, "Awp")
- case CSW_MP5NAVY:
- copy(name, imax, "Mp5navy")
- case CSW_M249:
- copy(name, imax, "M249")
- case CSW_M3:
- copy(name, imax, "M3")
- case CSW_M4A1:
- copy(name, imax, "M4a1")
- case CSW_TMP:
- copy(name, imax, "Tmp")
- case CSW_G3SG1:
- copy(name, imax, "G3sg1")
- case CSW_FLASHBANG:
- copy(name, imax, "闪光雷")
- case CSW_DEAGLE:
- copy(name, imax, "Deagle")
- case CSW_SG552:
- copy(name, imax, "Sg552")
- case CSW_AK47:
- copy(name, imax, "Ak47")
- case CSW_KNIFE:
- copy(name, imax, "野战匕首")
- case CSW_P90:
- copy(name, imax, "P90")
- }
- return 1
- }
- public clear_spr(parm[]) {
- new id = parm[0]
- new type = parm[1] // type 0=alive, 1=disconnect, 2=die in camping
- message_begin(MSG_ALL,SVC_TEMPENTITY)
- write_byte(125)
- write_byte(id)
- message_end()
- if (type == 2 && g_pl_dtp[id] && parm[2] == id) {
- new name_camper[32]
- get_user_name(id,name_camper,31)
- set_hudmessage(154, 20, 255, 0.17, 0.1, 0, 6.0, 10.0, 0.1, 0.15, 3)
- show_hudmessage(0,"上局 %s 蹲的太专心了, 一不留神把自己给蹲死了^n这局请时刻留意自己的蹲坑状态",name_camper)
- g_pl_dtp[id] = false
- }else if (type == 1) {
- set_hudmessage(154, 20, 255, 0.17, 0.1, 0, 6.0, 10.0, 0.1, 0.15, 3)
- show_hudmessage(0,"正在蹲坑的 %s 离开了服务器",parm[2])
- }else if (type == 0)
- show_camp_message(id,0)
- g_pl_spr[id] = false
- return PLUGIN_CONTINUE
- }
- public client_connect(id) {
- g_pl_camptimes[id] = 0
- g_pl_lim[id] = false
- g_pl_spr[id] = false
- campmeter[id] = 0
- return PLUGIN_CONTINUE
- }
- public client_disconnect(id) {
- if ((contain(cvarflags,"c") != -1) && (g_pl_spr[id] == true)) {
- new parm[34]
- parm[0] = id
- parm[1] = 1
- get_user_name(id,parm[2],31)
- set_task(0.5,"clear_spr",TASK_CAMP+id,parm,34)
- }
- if (contain(cvarflags,"d") != -1) {
- emit_sound(id,CHAN_VOICE,"player/breathe2.wav", 0.0, ATTN_NORM, SND_STOP, PITCH_NORM)
- }
- campmeter[id] = 0
- return PLUGIN_CONTINUE
- }
- public on_death(){
- new killer = read_data(1)
- new id = read_data(2)
- pausecounter[id]=true
- g_pl_dtp[id] = true
- if ((contain(cvarflags,"c") != -1) && (g_pl_spr[id] == true)) {
- new parm[3]
- parm[0] = id
- parm[1] = 2
- parm[2] = killer
- set_task(0.1,"clear_spr",TASK_CAMP+id,parm,3)
- }
- return PLUGIN_CONTINUE
- }
- public detect_enemy(id) {
- if (!is_user_alive(id))
- return PLUGIN_CONTINUE
- new myorigin[3],emorigin[3]
- new name[32],plist[32],pnum
-
- get_user_name(id,name,31)
- get_user_origin(id, myorigin)
- get_players(plist, pnum, "a")
- for(new i = 0; i < pnum; i++) {
- if (plist[i] == id)
- continue
- get_user_origin(plist[i], emorigin)
- new distance = get_distance(emorigin, myorigin)
- if ( float(distance) * 0.0254 < DETECT_RANGE ){
- if (get_user_team(id) == get_user_team(plist[i])){
- set_hudmessage(230, 180, 0, -1.0, 0.70, 0, 6.0, 6.0, 0.1, 0.15, 1)
- show_hudmessage(plist[i],"注意: 你的队友 %s 在与你距离 %.2f 米的地方蹲坑",name,float(distance) * 0.0254)
- client_print(plist[i],print_chat,"* 注意: 你的队友 %s 在与你距离 %.2f 米的地方蹲坑.",name,float(distance) * 0.0254)
- }else{
- set_hudmessage(230, 180, 0, -1.0, 0.70, 0, 6.0, 6.0, 0.1, 0.15, 1)
- show_hudmessage(plist[i],"注意: 有敌人在距离你 %.2f 米的地方蹲坑",float(distance) * 0.0254)
- client_print(plist[i],print_chat,"* 注意: 有敌人在距离你 %.2f 米的地方蹲坑.",float(distance) * 0.0254)
- }
- }
- }
- return PLUGIN_CONTINUE
- }
复制代码 |
本帖子中包含更多资源
您需要 登录 才可以下载或查看,没有账号?注个册吧
×
|